Cloud Function does a good job of redirecting all your print statements to logging. This is really good for basic work. However, for production ready code we need proper logging level with our application log. There are two way to achieve this. one way is to use cloud logging module provided by google and other is to write a custom formatter for python logging. I am going to share both the ways here.
Using Cloud Logging Module
For this you need to have google-cloud-logging python library in your requirement.txt
from google.cloud import logging as cloudlogging def hello_gcs(event, context): logger = get_logger(__name__) logger.info("info") logger.warn("warn") logger.error("error") logger.debug("debug") def get_logger(name): lg_client = cloudlogging.Client() lg_handler = lg_client.get_default_handler() cloud_logger = logging.getLogger("cloudLogger") cloud_logger.setLevel(logging.DEBUG) cloud_logger.addHandler(lg_handler) return cloud_logger
This is how it would look like, if you’ll use above code for cloud logging.
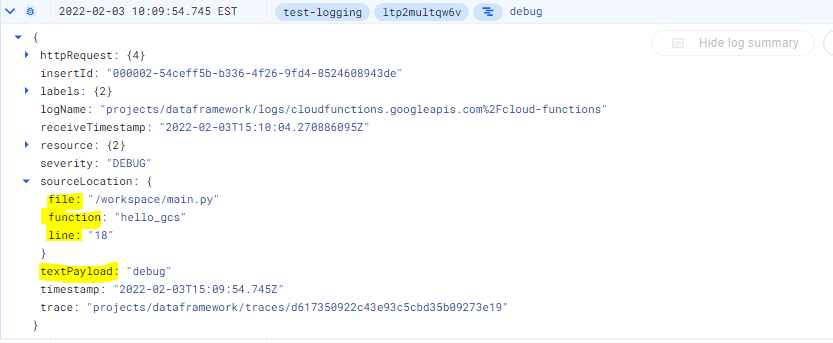
Using Custom Formatter
def hello_gcs(event, context): logger = get_custom_logger(__name__) logger.debug("debug") logger.info("info") logger.warn("warn") logger.error("error") class CloudLoggingFormatter(logging.Formatter): """Produces messages compatible with google cloud logging""" def format(self, record: logging.LogRecord) -> str: s = super().format(record) return json.dumps( { "message": s, "severity": record.levelname } ) def get_custom_logger(name): cloud_logger = logging.getLogger(name) handler = logging.StreamHandler(sys.stdout) formatter = CloudLoggingFormatter(fmt="[%(asctime)s] {%(filename)s:%(lineno)d} - %(message)s") handler.setFormatter(formatter) cloud_logger.addHandler(handler) cloud_logger.setLevel(logging.DEBUG) return cloud_logger
And this is how it looks with custom formatter.
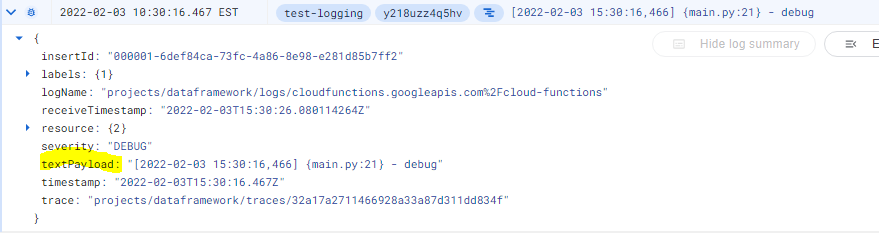
And this is the comparison for both,
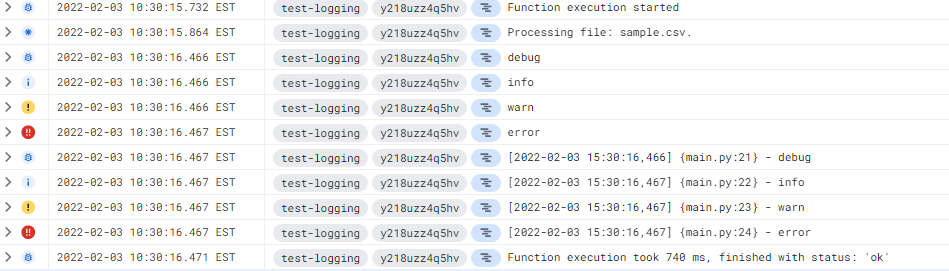
Be First to Comment